C# CONCEPTS
According to Gang of Four, abstract factory patterns can be assumed as the factory for creating factories.
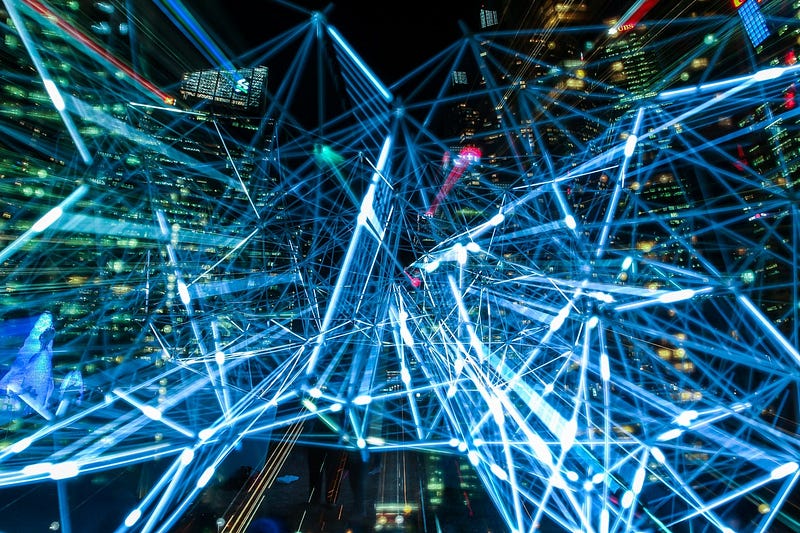
Learning Objectives
- What is the abstract factory design pattern?
- How to write code using the abstract factory design pattern?
- How to create a factory provider?
- How to create a client application(from the Main method) that uses factory provider
Prerequisites
Abstract factory pattern is purely an extension factory method; it’s recommended to go through the factory method before understanding abstract factory design.
https://singhsukhpinder.medium.com/factory-method-design-pattern-8994b9287265
- Basic knowledge of OOPS concepts.
- Any programming language knowledge.
The article demonstrates the abstract factory design pattern using the C# programming language. So, to begin with, C#
https://singhsukhpinder.medium.com/factory-method-design-pattern-8994b9287265
Getting Started
Let’s consider the same example of any Bank with account types as Savings and Current accounts. Now let’s implement the above example using the abstract factory design pattern.
Firstly, implement ISavingAccount and ICurrentAccount interfaces as follows:
public interface ISavingAccount{ }
public interface ICurrentAccount{ }
Inherit the interface in classes below
https://gist.github.com/ssukhpinder/183bf76ebea4620f622c63f04c72ae71
Let’s write an abstract class with abstract methods for each account type.
https://gist.github.com/ssukhpinder/1a999a263f76268c3ed37bed373300ae
Now, let’s create a factory implementation named “Bank1Factory,” which provides the implementation of abstract methods.
https://gist.github.com/ssukhpinder/91c9ca8d708f4e035b6d34197114c27d
The abstract factory design pattern differs from the factory method that it needs to implement a factory provider, which returns factories as per definition.
Now that we have all the abstractions and factories created. Let us design the factory provider. Please find below the code snippet for factory provider, where a static method will create a factory based upon account name.
https://gist.github.com/ssukhpinder/5e4caa76ba3d7df9ea84cbab3c3e07ff
How to use abstract factory provider?
Let’s take an example of a list of account numbers where if an account name consists of “B1” literal, then it will use Bank1Factory instance returned via factory provider.
https://gist.github.com/ssukhpinder/25db87d201e1c1b785d049e09fd66417
If the account name does not contain “B1” literal, then the program will output invalid {{accountName}}
Output
Please find below the output from the above code snippet.
Hello saving B1-456
Hello Current B1-456
Hello saving B1-987
Hello Current B1-987
Github Repo
https://singhsukhpinder.medium.com/factory-method-design-pattern-8994b9287265
Thank you for reading, and I hope you liked the article. Please provide your feedback in the comment section.