C# CONCEPTS
According to Gang of Four, the pattern adds extra responsibilities to a class object dynamically.
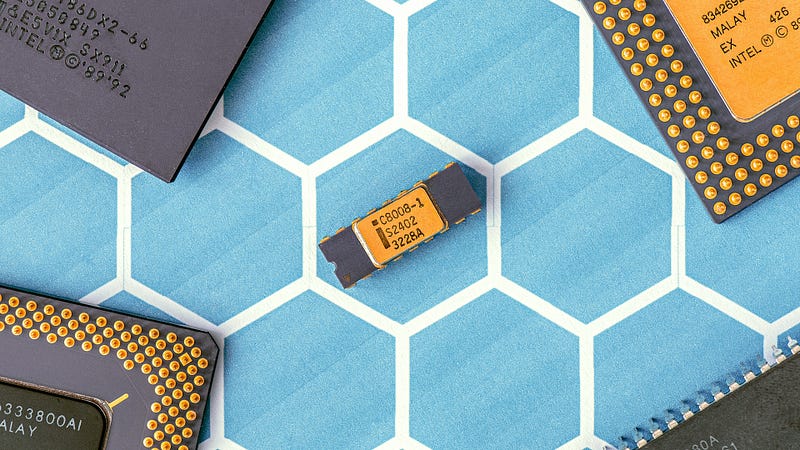
Use Case
Let’s consider the example of buying a car worth ten lakhs; the company provides the following additional features
- Sunroof
- Advance Music System
- and many more
With some additional features, the total price of the car increases. Let’s implement the above use case using Decorator Pattern.
Prerequisites
- Basic knowledge of OOPS concepts.
- Any programming language knowledge.
The article demonstrates the usage of decorator design patterns using the C# programming language. So, to begin with, C#
Learning Objectives
- How to code using a decorator design pattern?
Getting Started
Let us implement the use case defined above. Firstly define an abstract class Car and its base methods.
public abstract class Car{
public abstract int CarPrice();
public abstract string GetName();
}
Consider we a small car which extends above abstract class Car.
public class SmallCar : Car{
public override int CarPrice() => 10000;
public override string GetName() => "Alto Lxi";
}
Now implement the CarDecorator
class using the Car
component.
https://gist.github.com/ssukhpinder/001cc4870ee093cab1ac5eb2b6b44eaa
Now, let us create a separate class for each additional feature available for Car
inheriting the CarDecorator
class.
As per the use case, the additional features are a sunroof and an advanced music system.
AdvanceMusic.cs
Override the methods as
- Add the additional cost of an “advance music system” to the total car price.
- Update car name with additional feature name.
https://gist.github.com/ssukhpinder/95895731e860985d362e8c16aec49b26
Sunroof.cs
Override the methods as
- Add the additional cost of a “sunroof” to the total car price.
- Update car name with additional feature name.
https://gist.github.com/ssukhpinder/5421dba5d6ec0d2d0194fc85ec388375
Decorator Pattern in action
Create an instance of SmallCar
and output the name and price of the car.
Car car = new SmallCar();
Console.WriteLine($"Price of car {car.GetName()} : " + car.CarPrice());
Now, let’s add additional features as shown below
var car1 = new Sunroof(car);
var car2 = new AdvanceMusic(car);
Complete Code
https://gist.github.com/ssukhpinder/1f7f96843fe2a9c595a121eb292954ba
Output

Congratulations..!! You have successfully implemented the use case using the decorator pattern.
GitHub Sample Repo
Thank you for reading, and I hope you liked the article. Please provide your feedback in the comment section.
Follow me on
C# Publication, LinkedIn, Instagram, Twitter, Dev.to, Pinterest, Substack, Wix.